Required Initializers in Swift
Required initializers play a crucial role when subclassing classes. You can decorate initializers with a required keyword. Adding the required keyword assures that subclasses implement the required initializer.
You need the required keyword for two reasons —
- Factory methods
- Protocols
Factory Methods

The draw method accepts only String and returns an instance of Self. Self refers to the current type that the draw method is called on. this could be Shape class or one of its subclasses.
The reason that draw method returns Self is that Self is different for each subclass. If the method were to return a Shape instance, a draw wouldn’t be able to return an instance of Circle, Rectangle for example. But you’re not there yet. this gives the following error.

draw
is calling the init(shape:)
initializer on Shape or a Shape subclass. But how can the compiler be sure that a subclass will have an init(name:)
initializer? The required
designation will help the compiler to figure out it
The initializer throws an error because the draw method refers to self.init, it needs a guarantee that subclasses implement this method. Adding a required keyword to the designated initializer enforces subclasses to implement the initializer, which satisfies this requirement.
Solution :
First, you add the required keyword to the initializer in share method.


2. Protocol
Protocols are the second reason the required keyword exists.
When a protocol has an initializer, a class adopting that protocol must decorate that initializer with the required keyword. Let’s see why and how this works.
First, you introduce a protocol called NameDelegate, which contains an initializer.
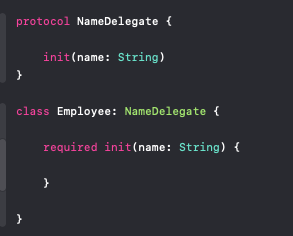
At this point, the compiler still isn’t happy. Because Employee conforms to the NameDelegate protocol, its subclasses also have to conform to this protocol and implement init(name: String).